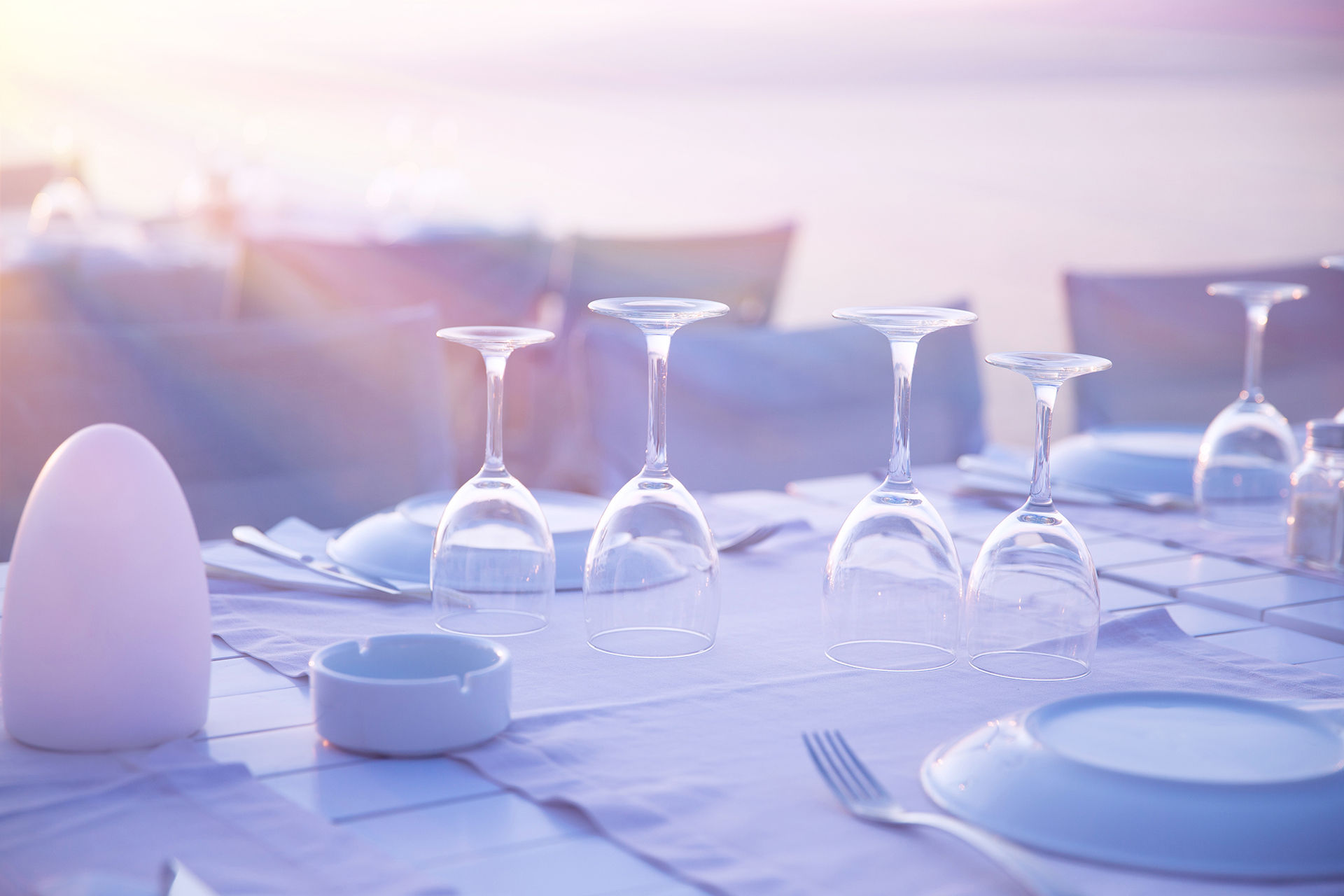
We're taking extra measures to ensure our final project will be finished as well. Learn More
Introduction
Processing is a flexible software sketchbook and a language for learning how to code within the context of the visual arts. Since 2001, Processing has promoted software literacy within the visual arts and visual literacy within technology. There are tens of thousands of students, artists, designers, researchers, and hobbyists who use Processing for learning and prototyping.
Processing continues to be an alternative to proprietary software tools with restrictive and expensive licenses, making it accessible to schools and individual students. Its free, liber, open-source status encourages community participation and collaboration which is vital to its growth. Contributors share programs, contribute code, and build libraries, tools, and modes to extend the possibilities of the software. The Processing community has written more than a hundred libraries for computer vision, data visualization, music composition, networking, 3D file exporting, and programming electronics.
Processing is used in classrooms worldwide, often in art schools and visual arts programs in universities, but it's also found frequently in high schools, computer science programs, and humanities curricula. In a National Science Foundation-sponsored survey, students in a college-level introductory computing course taught with Processing at Bryn Mawr College said they would be twice as likely to take another computer science class as the students in a class with a more traditional curriculum. The Processing approach has also been applied to electronics through the Wiring and Arduino projects. These projects use a modified version of the Processing programming environment to make it easier for students to learn how to program robots and countless other electronics projects.
​
Tutorials
Tutorial 1:
Mouse interaction demo: move the mouse to form a shape. Press the mouse button to invert the color.
Code:
void setup() {
size(640, 360);
noSmooth();
fill(126);
background(255);
}
void draw() {
if (mousePressed) {
stroke(255);
} else {
stroke(0);
}
line(mouseX-66, mouseY, mouseX+66, mouseY);
line(mouseX, mouseY-66, mouseX, mouseY+66);
}
Output:

Tutorial 2:
Keyboard: Click on the image to give it focus and press the letter keys to create forms in time and space. Each key has a unique identifying number. These numbers can be used to position shapes in space.
Code:
int rectWidth;
void setup() {
size(640, 360);
noStroke();
background(0);
rectWidth = width/4;
}
void draw() {
// keep draw() here to continue looping while waiting for keys
}
void keyPressed() { int keyIndex = -1;
if (key >= 'A' && key <= 'Z') {
keyIndex = key - 'A';
} else if (key >= 'a' && key <= 'z') {
keyIndex = key - 'a';
}
if (keyIndex == -1) {
// If it's not a letter key, clear the screen
background(0);} else { fill(millis() % 255);
float x = map(keyIndex, 0, 25, 0, width - rectWidth); rect(x, 0, rectWidth, height);
}
}

Output:

Output:
Tutorial 3:
radial-gradient: Draws a series of concentric circles to create a gradient from one color to another.
Code:
int dim;
void setup()
{ size(640, 360); dim = width/2; background(0);
colorMode(HSB, 360, 100, 100); noStroke(); ellipseMode(RADIUS);
frameRate(1); } void draw() { background(0);
for (int x = 0; x <= width; x+=dim) { drawGradient(x, height/2); } } void drawGradient(float x, float y)
{ int radius = dim/2;
float h = random(0, 360);
for (int r = radius; r > 0; --r)
{ fill(h, 90, 90);
ellipse(x, y, r, r); h = (h + 1) % 360; } }
Tutorial 4:
LoadDisplayImage: Images can be loaded
and displayed to the screen at their actual
size or any other size.
Code:
PImage img; // Declare variable "a" of type PImage void setup()
{ size(640, 360);
// The image file must be in the data folder of the current sketch
// to load successfully
img = loadImage("moonwalk.jpg");
// Load the image into the program }
void draw()
{
// Displays the image at its actual size at point (0,0) image(img, 0, 0);
// Displays the image at point (0, height/2) at half of its size
image(img, 0, height/2, img.width/2, img.height/2);
}
